React 1 - Hello React
This is the beginning of a more detailed introduction to building React based applications. For those looking for a super fast introduction refer to my other blogpost. Todays goal is to get a basic component up and running, transpile the JSX and ES6 and finally the the result in our browser.
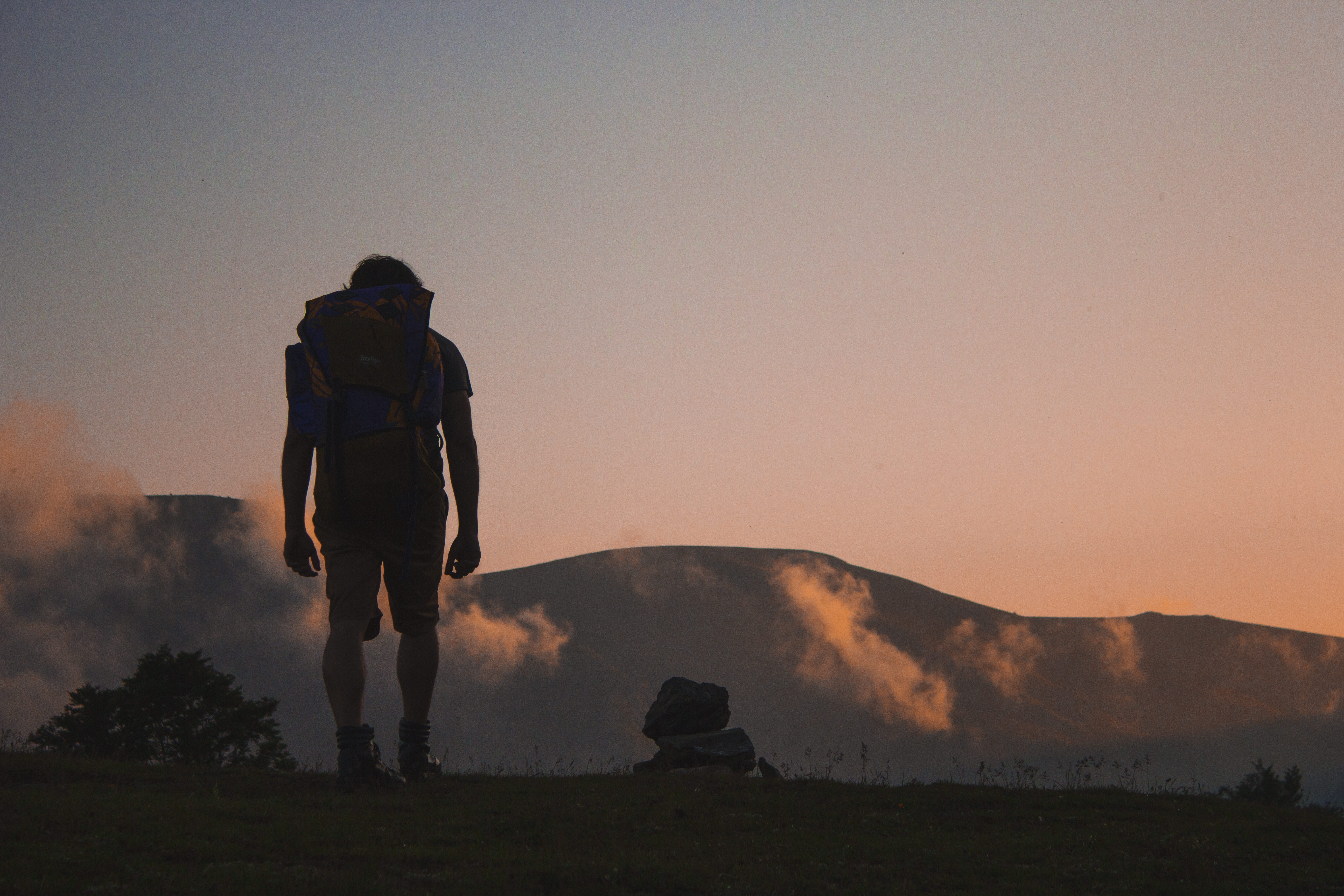
The base idea of React is simple enough. Describe how your application should look given a certain state. The beauty of this is how easily we can reason about it. No more thinking about how user input goes back into the system. We just describe how our site looks.
Just describe how your application looks, given a state.
Pre requireries
You will need Node installed on your system. I also strongly suggest to set up a package.json
file to keep organized and run scripts from. The rest of this article and series will assume that you do so.
You should also be familiar with ES6
Components
To organize things even more you can break your application down into so called components. They are a part of your application that might be used multiple times. Like a button or the way a users avatar is displayed. Lets take a look at a simple component.
//components/button.js
import React from 'react'
const button = ({ text, click }) => (
<button className="button" onClick={click}>
{text}
</button>
)
export default button
Lets take a look at this line for line. At the top we import React which you always need to do in all your components.
import React from 'react'
Then we define the button which is a function returning a JSX component and taking two arguments named text
and click
. We will later be able to pass properties with these names to our component. This is an ES6 function syntax using an implicit return.
const button = ({ text, click }) => <button></button>
Explicitly returning would also be possible and looks like this:
const button = ({ text, click }) => {
return <button></button>
}
Note that a component should always have a single outer wrapping element.
//Will throw an error
const button = ({text, click}) => (
<button></button>
<button></button>
)
//This will work okay
const button = ({text, click}) => (
<div>
<button></button>
<button></button>
</div>
)
JSX
JSX is a HTML like syntax you can use to describe your component. You can write plain HTML with custom tags and use {}
to add things, like render the text of a variable.
<button className="button" onClick={click}>
{text}
</button>
Notice that to add a class to our component we have to use className
instead of class
. Here we use {}
syntax to render the text of the button and add a function to be called when the button is clicked.
Entry point
Each React application needs an entry point. This file will wrap it all together and tell your application what to render. So lets set up to render some "Hello React" on our button.
import React from 'react'
import { render } from 'react-dom'
import Button from './components/button'
render(
<Button text="Hello React" click={() => alert('Hello React alert')} />,
document.querySelector('#app')
)
First we once again import React
and this time also render
which is a function render a React component into a given DOM element. Then we also import our Button
, by convention we capitalize components to distinguish between custom and native HTML tags in our JSX.
//index.js
import React from 'react'
import { render } from 'react-dom'
import Button from './components/button'
Further down we simply call render with our button and giving it some properties.
render(
<Button text="Hello React" click={() => alert('Hello React alert')} />,
document.querySelector('#app')
)
See the result
We are going to "compile" our application into a folder called build
. To actually see your application later create a build/index.html
file. Make sure it has an element with id app
and includes the script.
<!-- index.html -->
<div id="app">
</div>
<script src="js/main.js"></script>
We will be using Webpack to bundle our application together. You will need to install Webpack. Let's also install a local server with live reloading features so we don't have to refresh the browser to see our changes. While we are at it we should also pull in React and some stuff Webpack needs, we will go over all the packages.
npm install --save-dev react react-dom live-server webpack babel-core babel-loader babel-preset-es2015 babel-preset-react
| Package | Description | | ------------------- | --------------------------------------------------- | | react | The basic React package | | react-dom | rendering React components inside a DOM element | | live-server | A local server with live reloading of changed files | | webpack | Bundler for our application | | babel-core | Babel does the actual transpiling | | babel-loader | Enables Babel use in webpack | | babel-preset-es2015 | Transpiling of ES6 | | babel-preset-react | Transpiling of JSX to React |
So finally we can set up two script in our package.json
and run them both to see the results.
"webpack": "webpack --progress --colors --watch",
"serve": "live-server build"
Run them in separate consoles (use npm run webpack
) to have both going at the same time. Your browser will pop open and show you the resulting page. Any changes you make will trigger Webpack to bundle your application again and live-server to reload the page.
Wrap up
We now have a basic setup for React. Follow the next parts of this series when we look at composing components and hooking up Redux as a state manager.